I’ve been using Fluent SMTP for a while now for all the sites I build, and it works great. They have lots of connections, including popular email sending services like Mailgun.
However, what happens when for whatever reason your website can’t send any emails for one reason or another. It can’t send you an email telling you that, because well… it can’t send any emails. Fluent SMTP handles this well by allowing you to add a fallback email service, but it could be months before you realise that it’s been using the fallback email.
I regularly use Pushover to send native push notifications directly to my phone. So I thought I would combine this so that I am instantly notified by push notification when an email has failed to send.
1. Create our account
First we need to create our account. Pushover costs $5 (one time fee) per device you want to receive notifications.
2. Getting our tokens and keys
We will need 2 tokens. You can find these in the dashboard.
Your User Key
This is the identifier to send the notifications to you.
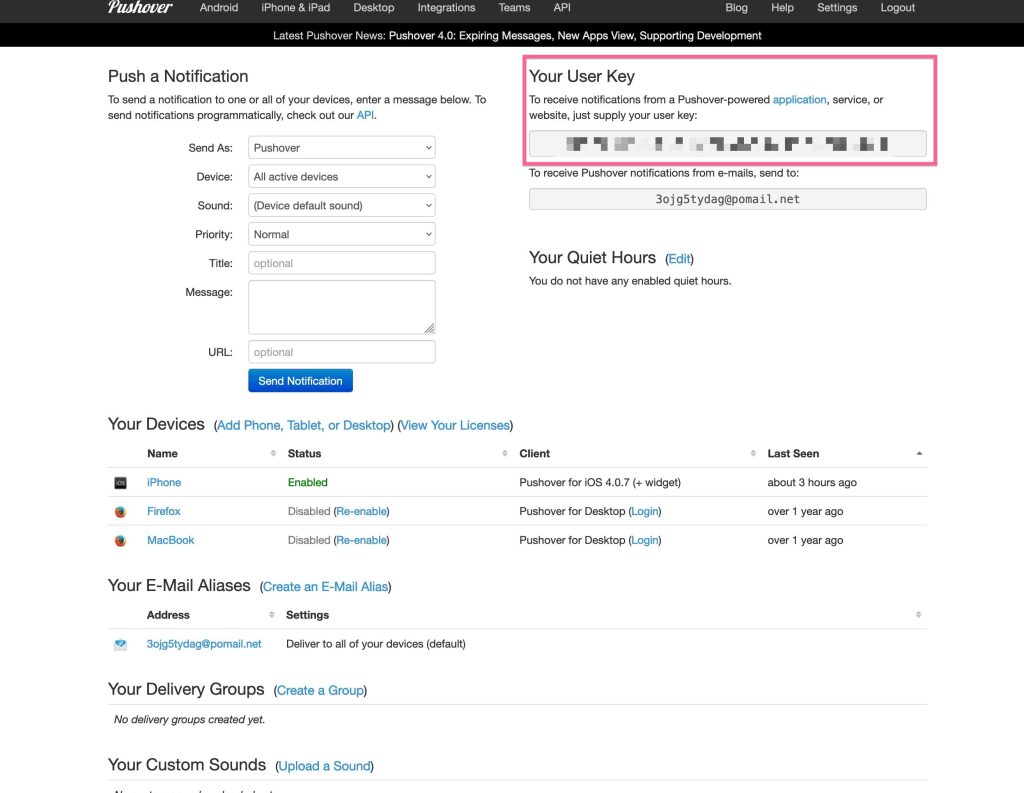
API Key
This allows Pushover to authenticate we have permission to send notifications.
Create a new application/API token. Make sure you label it something that makes sense for you as this is what will show up at the top of your push notification. You can also add an icon like your logo to make it easier to recognise when it comes through.
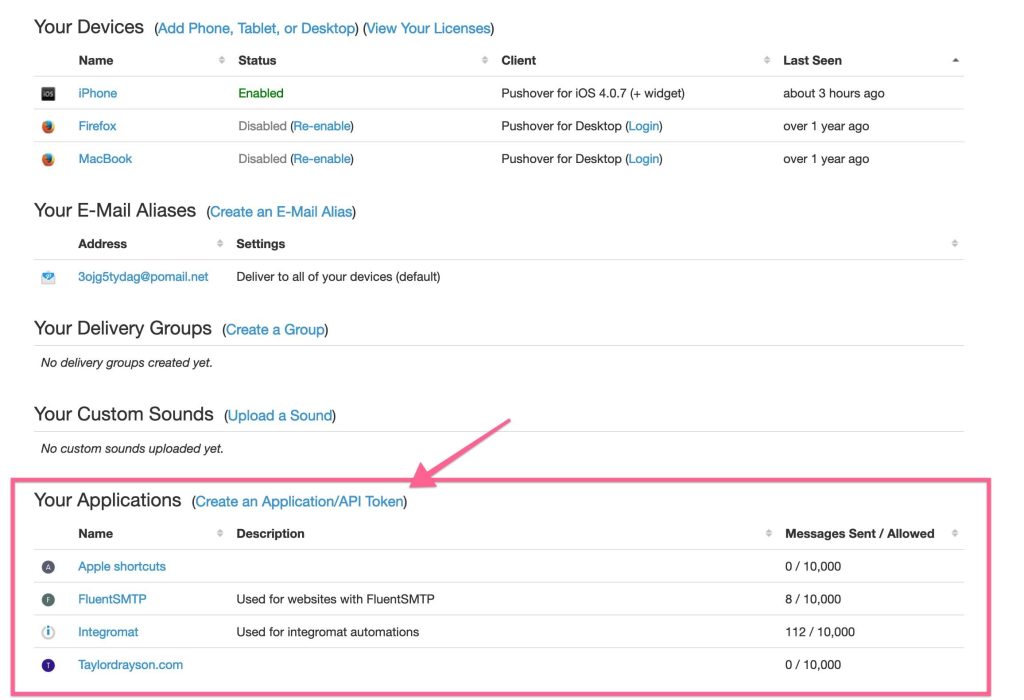
3. Adding our Pushover notification handler
Add the code below to functions.php
or code snippet plugin. Make sure to replace your $user_key
and $api_token
function send_pushover($data){ if(!isset($data['message'])) return; $api_token = 'XXXXXXXXXXXXXXX'; // Replace with your Pushover API token $user_key = 'XXXXXXXXXXXXX'; // Replace with your Pushover user key $url = 'https://api.pushover.net/1/messages.json'; $config_data = array( 'token' => $api_token, 'user' => $user_key, ); $data = array_merge($data, $config_data); $args = array( 'body' => $data, ); $response = wp_remote_post($url, $args); }
Copy
Code Breakdown
- Our function takes in an array with parameters for our API. Most of them are optional, however
message
is required. - We build our the body array to send to our
wp_remote_post
function which sends our notification.
4. Trigger notification on email fail
Now we want to call our send_pushover
function anytime an email fails to be delivered for whatever reason.
Add the code below to functions.php
or code snippet plugin.
/* * trigger_smtp_error * * @function Sends pushover for failed email sends * @author Taylor Drayson * @since 15/08/2023 */ add_action('wp_mail_failed', 'trigger_smtp_error', 10, 2); function trigger_smtp_error($response) { $data = [ 'message' => $response->get_error_message(), 'title' => get_bloginfo('name'), 'priority' => '1', 'sound' => '', 'url' => get_bloginfo('url'), 'url_title' => 'Open '. get_bloginfo('name') .' website' ]; send_pushover($data); }
Copy
Code Breakdown
- We call the action hook
wp_mail_failed
- Next we create our message which returns the error
- Set the priority to 1 which is high priority
- Set the title to the name of the website
- Add the link to the website
You can find a list of all the possible API parameters here.
Adding other integrations
If you want to add other integrations with webhooks or other services.
You can use the same action hook above. I’ve added some useful variables to help you get started.
add_action('wp_mail_failed', 'trigger_smtp_error', 10, 2); function trigger_smtp_error($response) { $error = $response->get_error_message(); $site_title = get_bloginfo('name'); $site_url = get_bloginfo('url'); // Do your custom integration here }
Copy
Conclusion
If everything is done correctly, you should now receive a push notification whenever an email fails to send on your website.
This can be copied and added across all your websites, and it will dynamically populate the name and URL of the website along with the correct error message.